[MasterThe Coding Interview]Graphs
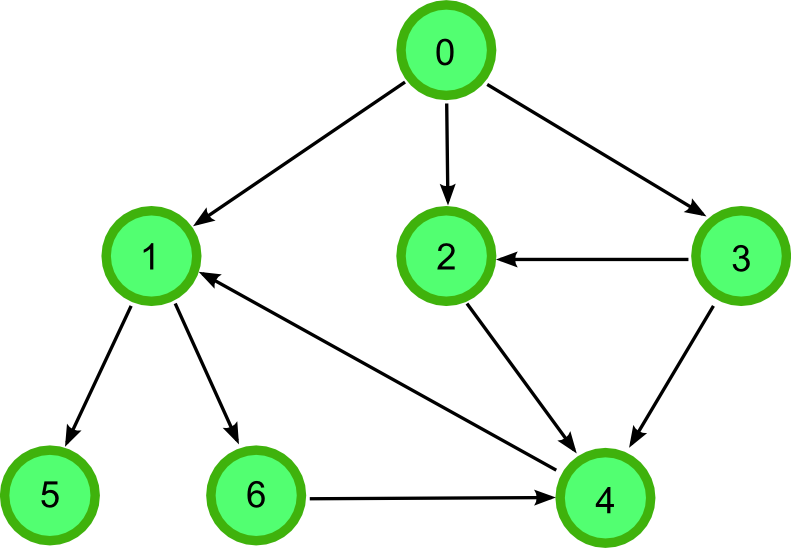
本篇為MasterThe Coding Interview教學影片筆記文
由N個nodes和M條links組合而成的資料結構就叫做Graphs,Linked list是一種Graph,Tree也算是一種Graphs。
Graphs分成有向和無向的,undirected像是facebook的好友功能,當你和一個人成為好友的時候,你們是互相為好友,directed像是instagram的追蹤功能,當你追蹤一個帳號的時候,是單方面的,他不一定要追蹤你。
Graphs可以分為有權重和無權重的,當圖的link都有權重的時候,我們就可以來算最短路徑的,像是google map的最佳路徑功能。
Graphs可以分為Cyclic和Acyclic,有環和無環的圖形。
Graphs的資料可以利用以下3種方法來記錄:
1 | //Edge List |
Implement an Graphs
1 | class Graph { |
When can use arry?
優點 | 缺點 |
---|---|
Relationships | Scaling is hard |